How to Add Page Transition Animation in React Router with Framer Motion
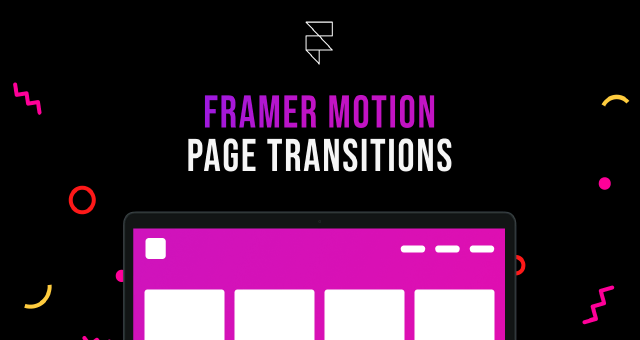
GX Anshu | Jul 12, 2023
First, we need to install the framer-motion
and react-router-dom
libraries. We can do this with the following commands:
npm install framer-motion
npm install react-router-dom
Next, we need to create a new React app. We can do this with the following command:
npx create-react-app framer-motion-page-transitions
Once the app is created, we need to add the AnimatePresence
component to the App.js
file. This component will ensure that our animations are only rendered when they’re visible.
import React from "react";
import { HashRouter, Routes, Route } from "react-router-dom";
import { AnimatePresence } from "framer-motion";
export default function App(): JSX.Element {
return (
<HashRouter>
<AnimatePresence mode="wait">
<Routes>
<Route path="/" element={<Home />} />
{/*add more route here*/}
</Routes>
</AnimatePresence>
</HashRouter>
);
}
Creating the Animation
Now, we need to create the animation. We’ll do this by creating a new component called AnimatedView.tsx
. This component will take a child component and add an animation to it.
import { LazyMotion, m, domAnimation } from "framer-motion";
export default function AnimationPage({ children }: any): JSX.Element {
return (
<LazyMotion features={domAnimation}>
<m.div
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
exit={{ opacity: 0 }}
transition={{ duration: 0.5 }}
>
{children}
</m.div>
</LazyMotion>
);
}
This code will create an animation that fades the child component in and out over a duration of 0.5 seconds.
Wrapping the Pages
The final step is to wrap all of our pages in the AnimatedView
component. We can do this by updating the Home.js
file to the following:
import React from "react";
import { AnimationPage } from "AnimatedView";
export default function Home(): JSX.Element {
return <AnimationPage>{/*code for home page*/}</AnimationPage>;
}
Now, when we navigate to different pages in our app, they will be animated in and out.
Conclusion
That’s it! We’ve now learned how to add page transition animation in React Router with Framer Motion in very low JavaScript. By using the LazyMotion
component, we were able to reduce the size of the JavaScript for more than 50%.